1. Create a new project EchoService in Visual Studio based on "WCF Service Library" template.
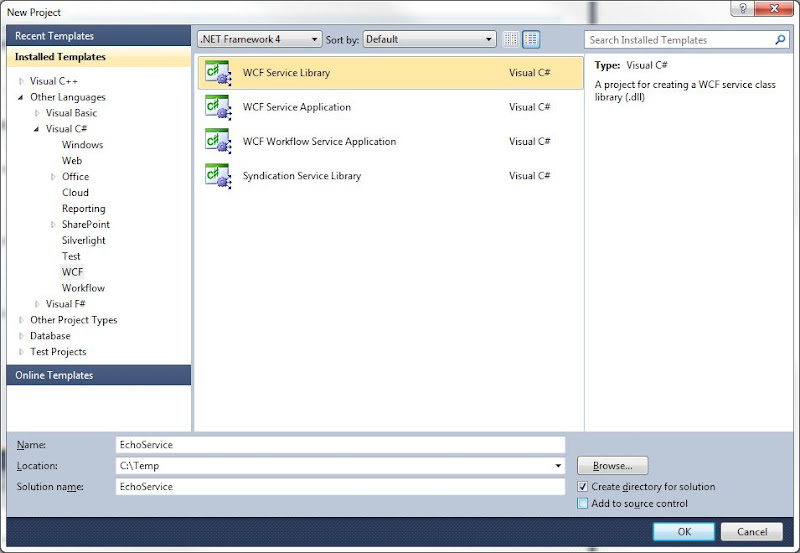
2. Go to the project properties and change target framework to ".NET Framework 4".
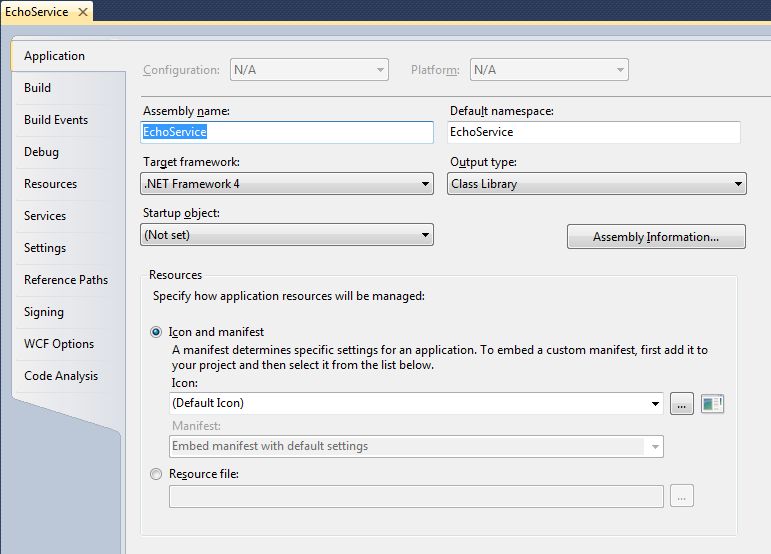
3. Open App.config file and do the following changes:
- change base address to http://localhost:8000/
- replace endpoint section to
<endpoint address="" behaviorConfiguration="webBehavior" binding="webHttpBinding" contract="EchoService.IService1">
- add the following code under behaviors section:
<endpointBehaviors>
<behavior name="webBehavior">
<webHttp/>
</behavior>
</endpointBehaviors>
4. Open IService1.cs file and replace it's content with the following code:
using System; using System.ServiceModel; using System.ServiceModel.Web; using System.IO; namespace EchoService { [ServiceContract] public interface IService1 { [WebInvoke(UriTemplate = "echo")] Stream HandleMessage(Stream request); } }
5. Open Service1.cs file and replace it's content with the following code:
using System; using System.ServiceModel; using System.ServiceModel.Channels; using System.IO; using System.ServiceModel.Web; namespace EchoService { public class Service1 : IService1 { public Stream HandleMessage(Stream request) { StreamReader reader = new StreamReader(request); string text = "EchoServer received: " + reader.ReadToEnd(); System.Text.ASCIIEncoding encoding = new System.Text.ASCIIEncoding(); MemoryStream ms = new MemoryStream(encoding.GetBytes(text)); WebOperationContext.Current.OutgoingResponse.ContentType = "text/html"; return ms; } } }
6. Start debugging. If everything OK then Visual Studio starts WCF Test Client. Now you can open you favorite test tool (I use Fiddler) and send a post request to http://localhost:8000/echo.
7. In my case I sent a word "test" as request and received back "EchoServer received: test".
Enjoy.
How did you add in test to the body?
ReplyDeleteDo you mean in Fiddler?
ReplyDeleteHow would I use this with say Twilio.com to recieve a SMS and use it in an application. I assume this could be used to grab the POST method which contains the SMS details. TIA!
ReplyDelete-Rob